Boost Your React App with a Sleek Command Palette Using cmdk
If you have ever used macOS Spotlight or the VS Code Command Palette, you know how powerful it feels to summon a search-driven interface with a simple keyboard shortcut.
It’s fast, it’s accessible, and it saves users from getting lost in endless menus.
Now, imagine you could build that same magical experience into your own React applications — and without spending hours handling keyboard navigation, accessibility quirks, or filtering logic.
Enter cmdk
, a brilliant lightweight library that brings command palettes to your app in a highly customizable and accessible way.
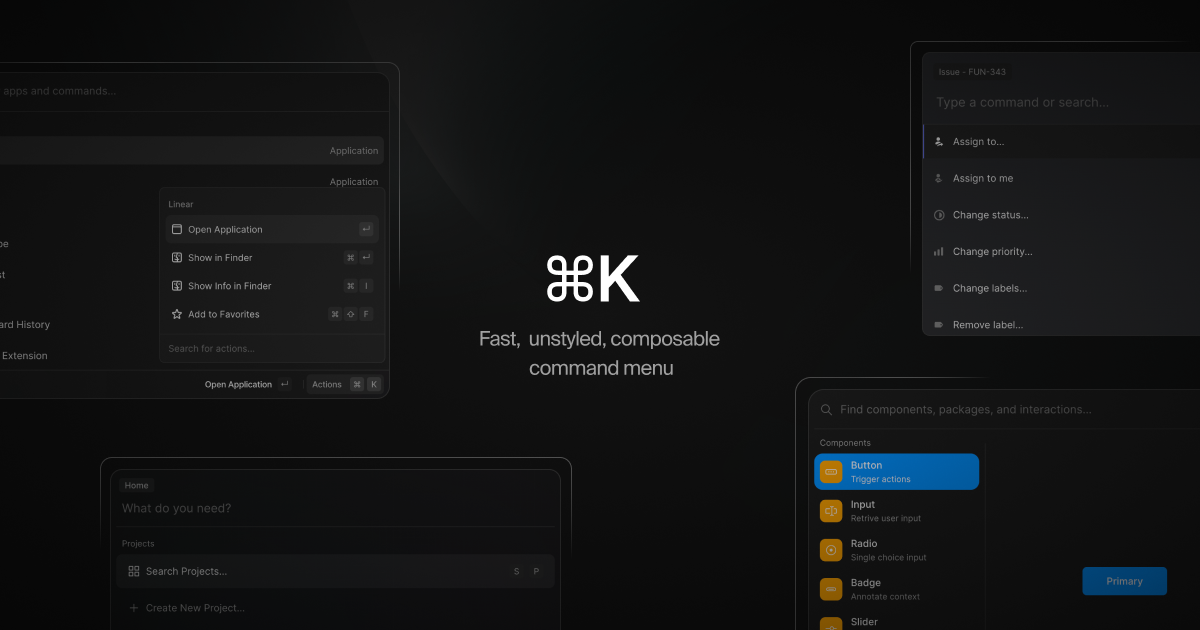
What Exactly is cmdk
?
cmdk
(short for Command Menu Kit) is a headless React component library that helps you build command menus quickly.
Unlike fully styled component libraries, cmdk
gives you the logic and accessibility out of the box, but leaves styling and layout completely up to you.
This means you get maximum flexibility: whether you want a minimalist look or a fully branded experience, you're in full control.
Why Should You Care About a Command Palette?
Command palettes are becoming a must-have UX feature in modern applications:
- Fast navigation without memorizing where everything is.
- Enhanced discoverability of features.
- Keyboard power users love it.
- Mobile-friendly designs can also benefit with touch-compatible implementations.
Products like Linear, Vercel Dashboard, and Raycast have made command palettes almost expected in high-quality software.
If you want to level up your app’s UX, a command menu is no longer a luxury — it’s a competitive edge.
Core Features of cmdk
Here’s why cmdk
stands out:
- 🛠 Headless and Unstyled — You control every pixel.
- ⚡ Blazing Fast — Optimized for React with zero bloat.
- 🎯 Accessible by Default — Built-in ARIA roles, keyboard navigation.
- 🔍 Live Filtering — Instant search as you type.
- 🔗 Composable API — Create groups, separators, modals easily.
Basic Example: Your First Command Menu
Let’s start simple. Here’s how you can build a minimal command palette with cmdk
:
import { Command } from 'cmdk';
function CommandMenu() {
return (
<Command label="Global Command Menu">
<Command.Input placeholder="Type a command..." />
<Command.List>
<Command.Item>Profile</Command.Item>
<Command.Item>Settings</Command.Item>
<Command.Item>Logout</Command.Item>
</Command.List>
</Command>
);
}
✨ That’s it! cmdk
handles all the focus management, keyboard navigation, and search filtering behind the scenes.
Taking It Further: Adding a Modal and Hotkey
Usually, you want your command palette to appear when pressing Cmd+K (or Ctrl+K on Windows). Here’s a more real-world example:
import { useState, useEffect } from 'react';
import { Command } from 'cmdk';
export default function CommandPalette() {
const [open, setOpen] = useState(false);
useEffect(() => {
const down = (e: KeyboardEvent) => {
if (e.key === 'k' && (e.metaKey || e.ctrlKey)) {
e.preventDefault();
setOpen((open) => !open);
}
};
window.addEventListener('keydown', down);
return () => window.removeEventListener('keydown', down);
}, []);
if (!open) return null;
return (
<div className="fixed inset-0 bg-black bg-opacity-50 flex items-center justify-center">
<Command className="bg-white rounded-md w-80 p-4 shadow-lg">
<Command.Input placeholder="Type a command..." />
<Command.List>
<Command.Item onSelect={() => alert('Profile clicked')}>Profile</Command.Item>
<Command.Item onSelect={() => alert('Settings clicked')}>Settings</Command.Item>
<Command.Item onSelect={() => alert('Logout clicked')}>Logout</Command.Item>
</Command.List>
</Command>
</div>
);
}
🧠 Explanation:
- Press
Cmd+K
orCtrl+K
to toggle the palette. - A simple
div
with a dark overlay appears with theCommand
inside. - Items respond to selection with an alert — in real apps, you would navigate or trigger actions.
Illustration: How It Feels
User presses Cmd+K ➔
A small modal appears ➔
User types "Set..." ➔
Settings item is immediately filtered ➔
Arrow down + Enter ➔
Settings page opens instantly
Just like VS Code, Linear, or Slack’s quick switcher — but inside your own app.
Seamless, fast, and smooth.
Things to Keep in Mind
Here are some considerations when using cmdk
:
- State management:
You will need to handle the open/close state manually (usually withuseState
). - Styling:
cmdk
doesn’t come with default CSS, so you should style it yourself using TailwindCSS, vanilla CSS, or your favorite method. - Accessibility:
Whilecmdk
provides ARIA roles, make sure your modal and background overlay are also accessible (e.g., trapping focus when open). - Grouping commands:
You can use<Command.Group>
to separate related items nicely. - Performance:
If you have thousands of commands, you may want to paginate or virtualize the list.
Finally
Adding a command palette is one of the highest-leverage UX improvements you can make — and with cmdk
, it’s no longer a massive engineering task.
You get keyboard shortcuts, search-driven navigation, and instant interactions — all while keeping full creative control over how it looks and feels.
If you want your app to feel like the future, start experimenting with cmdk
. 🚀
Comments ()