Enhancing Data Variability: Randomly Updating the views Column in MySQL
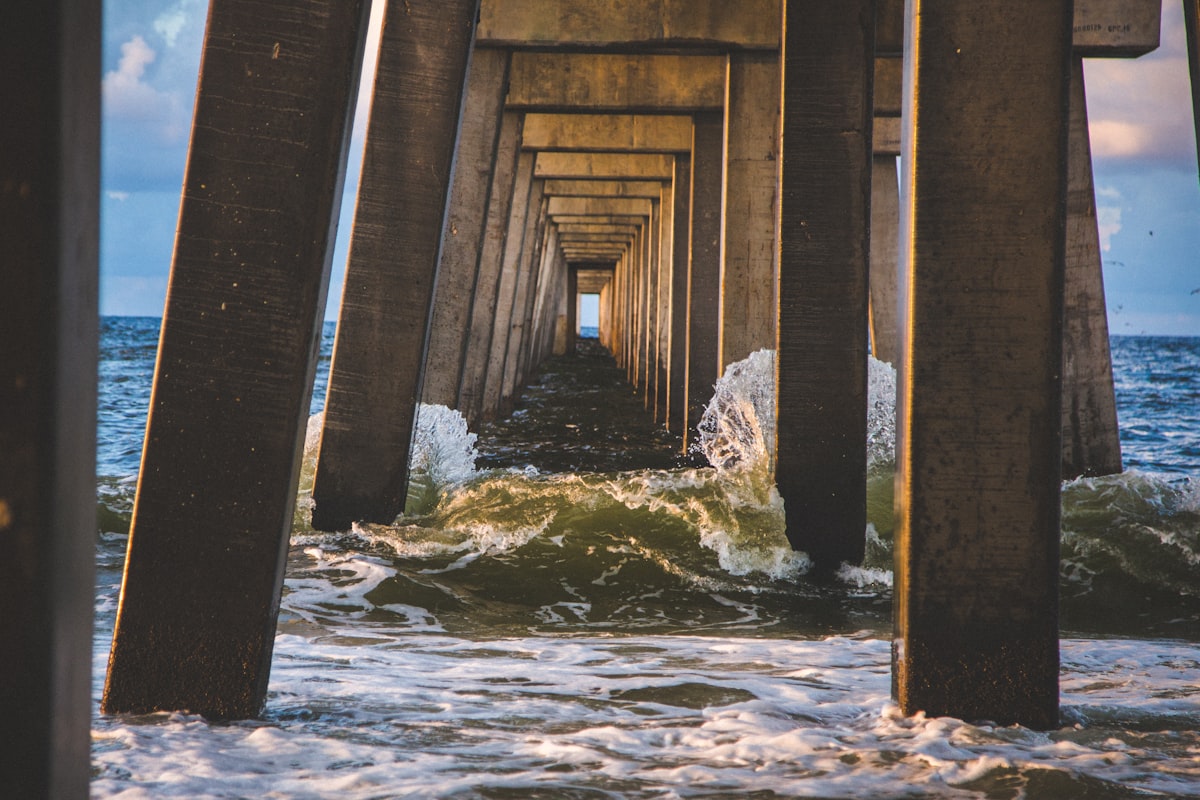
In database management and software development, there often arises a need to update data dynamically. One such instance is when you want to simulate realistic data for analytics or testing purposes. In this article, we'll explore how to randomly update the views
column in a MySQL table named shorts
with values ranging from 1000 to 9999. We'll also discuss several important considerations and potential extensions to this approach.
The Basic SQL Update Statement
To achieve this, you can use the MySQL UPDATE
statement along with a mathematical function to generate random values. The SQL statement below demonstrates how to perform this update:
UPDATE shorts
SET views = FLOOR(1000 + RAND() * 9000);
Breaking Down the Query
RAND()
Function:
This function generates a random decimal value between 0 (inclusive) and 1 (exclusive). It is the core element that introduces randomness into our update.- Scaling the Value:
By multiplyingRAND()
by 9000, the resulting value scales from 0 to almost 9000. This scaling allows us to spread the possible results over a desired range. - Shifting the Range:
Adding 1000 shifts the entire range upward. Without the addition, the minimum value would be 0, but with the shift, the range becomes 1000 to 9999.999.... - Rounding with
FLOOR()
:
TheFLOOR()
function ensures that the random decimal is converted into an integer. Hence, the result is always within the integer range of 1000 to 9999.
Considerations and Best Practices
When using this approach, there are several points you might need to consider depending on your project's requirements.
Scope of Update
Entire Table vs. Subset:
The provided query updates all rows in the shorts
table. If you need to update only a subset of rows, add a WHERE
clause to specify the criteria. For example:
UPDATE shorts
SET views = FLOOR(1000 + RAND() * 9000)
WHERE <condition>;
Replace <condition>
with your specific condition, such as updating only records created after a certain date.
Transaction Safety
- Batch Updates:
For larger tables, consider updating in batches to avoid locking the table for too long and to reduce the impact on database performance. This is particularly useful in high-traffic production environments. - Backup:
Always perform a backup before executing mass updates, especially if random values can impact business-critical metrics or analytics.
Randomness Consistency
- Reproducibility:
If your application requires reproducibility (e.g., in tests), be aware that theRAND()
function generates a new random number each time. In such cases, consider setting a seed forRAND()
if your environment supports it. - Security Considerations:
Although using random numbers in this context is generally safe, be cautious when integrating randomness in security-related operations, where predictability could be a concern.
Testing the Update
- Small Scale Testing:
Before running the update on the entire dataset, try it on a few rows or a test table to ensure that the randomization behaves as expected. This reduces the risk of unintended updates.
Alternative Approaches
- Stored Procedures:
For more complex randomization logic or additional processing, consider using a stored procedure that encapsulates the random update logic. This can improve maintainability and readability in complex systems. - Logging Changes:
In some use cases, it might be beneficial to log the previous values before updating, either in a backup table or through an audit trail, allowing you to track changes made by the random update process.
Finally
Updating the views
column in a MySQL table to a random value within a specific range is a straightforward process that can bring versatility and realism to your data. By utilizing the RAND()
, scaling, shifting, and FLOOR()
functions, you can easily generate random integers within any desired range.
Remember to consider the scope of your update, ensure transaction safety, and validate your approach in a controlled environment before applying it widely. Incorporating these considerations will help maintain the integrity and performance of your database, while also providing flexible and dynamic data updates.
By understanding both the technical implementation and the practical implications, you can leverage random data updates as a powerful tool in your database management and development toolkit.
Comments ()