Understanding and Avoiding the Dangers of Dangling Pointers in C
When working with C, one of the key challenges developers face is managing memory manually. While this gives you fine-grained control over how your program allocates and deallocates memory, it also introduces potential risks, such as dangling pointers. This article dives into what dangling pointers are, why they are dangerous, and how to mitigate their risks effectively.
What Are Dangling Pointers?
A dangling pointer occurs when a pointer continues to hold the memory address of a block of memory that has already been deallocated (freed). Once the memory is freed, accessing or modifying it through the pointer results in undefined behavior, meaning that your program might:
- Seem to work correctly.
- Crash unexpectedly.
- Produce incorrect or unpredictable results.
For example:
#include <stdio.h>
#include <stdlib.h>
int main() {
int* ptr = malloc(sizeof(int)); // Allocate memory
*ptr = 42; // Assign a value
printf("Value: %d\n", *ptr);
free(ptr); // Free the memory
*ptr = 50; // Dangling pointer access
printf("Value: %d\n", *ptr); // Undefined behavior
return 0;
}
On some systems, the code may run without issue, producing the output:
Value: 42
Value: 50
However, this behavior is not guaranteed. It depends entirely on how the system and runtime manage freed memory. This unpredictability is what makes dangling pointers so dangerous.
Why Undefined Behavior Can Be Tricky
Undefined behavior is the hallmark of problems like dangling pointers. Here’s why it’s tricky:
- System-Dependent Behavior: On some systems, accessing freed memory will crash your program immediately (e.g., segmentation fault). On others, it might appear to work because the memory hasn’t been reused yet.
- Compiler Optimizations: Compilers might optimize your code in ways that exacerbate undefined behavior. For example, enabling higher optimization levels (
-O2
,-O3
) in GCC might make your program behave differently. - Debugging Challenges: Since the behavior depends on runtime conditions, debugging dangling pointer issues can be like chasing ghosts, especially in large and complex programs.
How to Mitigate Dangling Pointer Issues
Here are practical steps to avoid or handle dangling pointers:
1. Set Pointers to NULL After Freeing
Once you free a pointer, immediately set it to NULL
. A NULL pointer is easier to detect and prevents accidental access:
free(ptr);
ptr = NULL; // Now ptr doesn't point to freed memory.
2. Avoid Reusing Freed Memory
Reusing memory directly after freeing it can lead to serious bugs. Instead of trying to "reclaim" freed pointers, rely on memory allocators like malloc
or calloc
for fresh allocations.
3. Use Tools to Detect Memory Issues
Modern compilers and tools can help you identify dangling pointer issues:
- AddressSanitizer: A powerful memory error detector available in GCC and Clang. Compile your program with
-fsanitize=address
to catch issues like heap-use-after-free. - Valgrind: A tool for memory debugging that can detect memory leaks and dangling pointer access.
For example, compiling with AddressSanitizer:
gcc -g -fsanitize=address -o main main.c
./main
Might produce output like:
AddressSanitizer: heap-use-after-free
On mine like this.
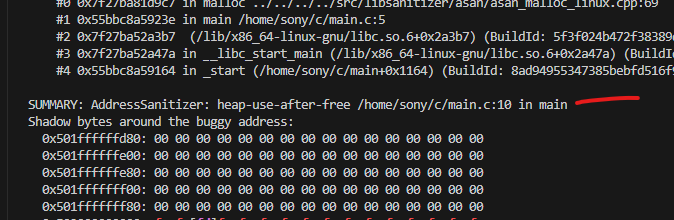
4. Minimize Manual Memory Management
Consider using higher-level memory management techniques or libraries where possible. In C++, for instance, smart pointers like std::unique_ptr
or std::shared_ptr
help avoid dangling pointers by managing the lifetime of memory automatically.
Other Considerations
1. Double-Free Errors
A related issue is double-free errors, which occur when you call free()
on the same memory block multiple times. This can corrupt your memory allocator and crash your program:
int* ptr = malloc(sizeof(int));
free(ptr);
free(ptr); // Double free: dangerous!
2. Memory Leaks
While avoiding dangling pointers, be careful not to forget freeing memory altogether. A memory leak happens when you lose all references to allocated memory without freeing it, leading to increased memory usage over time.
3. Accessing Freed Memory Through Aliased Pointers
Even if one pointer is set to NULL
, other pointers referencing the same memory can still cause problems:
int* ptr1 = malloc(sizeof(int));
int* ptr2 = ptr1;
free(ptr1);
ptr2 = NULL; // ptr2 still dangles!
Finally
While the output of the dangling pointer example might appear harmless, relying on undefined behavior is a recipe for disaster. Always clean up pointers properly, use tools to detect errors early, and prefer safer programming practices wherever possible. By understanding and addressing these risks, you can write more robust and reliable C programs.
Remember: Undefined behavior is unpredictable and dangerous. The best defense is prevention.
Comments ()