Understanding SQL Joins: A Comprehensive Guide to Combining Data
When working with relational databases, SQL joins are essential tools for combining data from multiple tables based on a related column. Joins allow you to create more meaningful queries by linking tables that store data in a structured way. While SQL provides different types of joins, each serves a specific purpose. In this article, we'll break down the most commonly used joins and explore other considerations to help you understand how to leverage them effectively.
1. INNER JOIN: The Foundation of Joins
The INNER JOIN is one of the most commonly used joins. It retrieves records that have matching values in both tables. If there is no match between the two tables, those rows will be excluded from the result set. This makes it ideal for situations where you need to combine only the data that exists in both tables.
SQL Query:
SELECT *
FROM A
INNER JOIN B ON A.key = B.key;
- Explanation: This query returns all rows from A and B where the values of
A.key
andB.key
are equal. If no match is found, the row is excluded. - Use Case: You might use an inner join when working with two tables like customers and orders, where you want to find only those customers who have placed orders.
2. FULL OUTER JOIN: All Rows, Matched or Not
The FULL OUTER JOIN returns all rows from both tables, with matching rows from both sides where available. If there's no match, the result will contain NULL values for the columns of the table that doesn't have a matching row.
SQL Query:
SELECT *
FROM A
FULL JOIN B ON A.key = B.key
WHERE A.key IS NULL OR B.key IS NULL;
- Explanation: This query retrieves all rows where there is either a match in both tables or no match at all. The
WHERE
clause is added to filter out the matched rows, leaving only the non-matching ones from each table. - Use Case: Full outer joins are useful when you want to capture all possible data, regardless of whether a match exists. For example, if you are merging product catalogs from two different suppliers and want to see all the products from both suppliers, even if some items are not common to both.
3. LEFT JOIN: Everything from the Left Table
A LEFT JOIN (or LEFT OUTER JOIN) returns all rows from the left table (in our case, A), and the matched rows from the right table (B). If no match is found, the result will contain NULL values for columns from the right table.
SQL Query:
SELECT *
FROM A
LEFT JOIN B ON A.key = B.key
WHERE B.key IS NULL;
- Explanation: This query retrieves all rows from A and the matching rows from B. If there’s no match in B, the query still returns the row from A with
NULL
for B’s columns. TheWHERE
clause filters out rows where B has a match and only keeps the rows where B’s key isNULL
. - Use Case: Left joins are typically used when you want to retain all records from the left table. For instance, when working with an employees table and a departments table, and you need to see all employees, even if they aren't assigned to a department.
4. RIGHT JOIN: Everything from the Right Table
The RIGHT JOIN (or RIGHT OUTER JOIN) works similarly to the left join but retrieves all rows from the right table (B) and the matching rows from the left table (A). If there’s no match in A, the result contains NULL
values for columns from A.
SQL Query:
SELECT *
FROM A
RIGHT JOIN B ON A.key = B.key
WHERE A.key IS NULL;
- Explanation: This query returns all rows from B, and if there’s no corresponding match in A, it returns
NULL
for the columns from A. TheWHERE
clause filters out the matched rows, showing only those where there is no match in A. - Use Case: Right joins are useful when you want to capture all records from the right table, such as when dealing with orders and products, and you want to see all products, even those that haven’t been ordered.
Other Types of Joins You Might Need
While INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN are the most common, there are a few more joins you should be aware of:
5. CROSS JOIN: Cartesian Product
A CROSS JOIN returns the Cartesian product of two tables, meaning it returns every combination of rows from both tables. This join doesn't require any condition.
SQL Query:
SELECT *
FROM A
CROSS JOIN B;
- Explanation: Every row from A is combined with every row from B. If A has 3 rows and B has 4, the result will contain 12 rows.
- Use Case: CROSS JOIN is rarely used but can be useful for generating combinations of rows, such as creating a list of all possible combinations of products and colors.
6. SELF JOIN: Joining a Table with Itself
A SELF JOIN is a regular join, but the table is joined with itself. This is particularly useful when you need to relate rows within the same table.
SQL Query:
SELECT A.*, B.*
FROM Employees A
JOIN Employees B ON A.manager_id = B.id;
- Explanation: In this case, an employee (A) is joined with their manager (B), both coming from the same Employees table.
- Use Case: Self joins are useful for hierarchical data, like when dealing with organizational charts or family trees.
Key Considerations When Using Joins
- Performance: Joins can be expensive in terms of performance, especially when dealing with large tables. Make sure your join conditions are properly indexed to speed up query execution.
- Null Values: When using outer joins (left, right, or full), be aware of
NULL
values, which can sometimes lead to confusion or unexpected results. Proper handling ofNULL
values in your logic is essential. - Join Type Selection: Always choose the right type of join based on the business logic you're implementing. For example, if you need only matching records, use INNER JOIN. If you need all data from one table, regardless of matching, consider LEFT JOIN or RIGHT JOIN.
- Multiple Joins: You can join multiple tables in a single query. However, this can quickly increase the complexity of your SQL statement, so make sure you're clear on the relationships between tables to avoid errors.
- Aliases for Readability: When working with multiple joins, using table aliases (e.g.,
A
,B
) makes the query more readable and helps prevent ambiguity, especially in large datasets.
Need Visual Cheat Sheet?
This can be very handy.
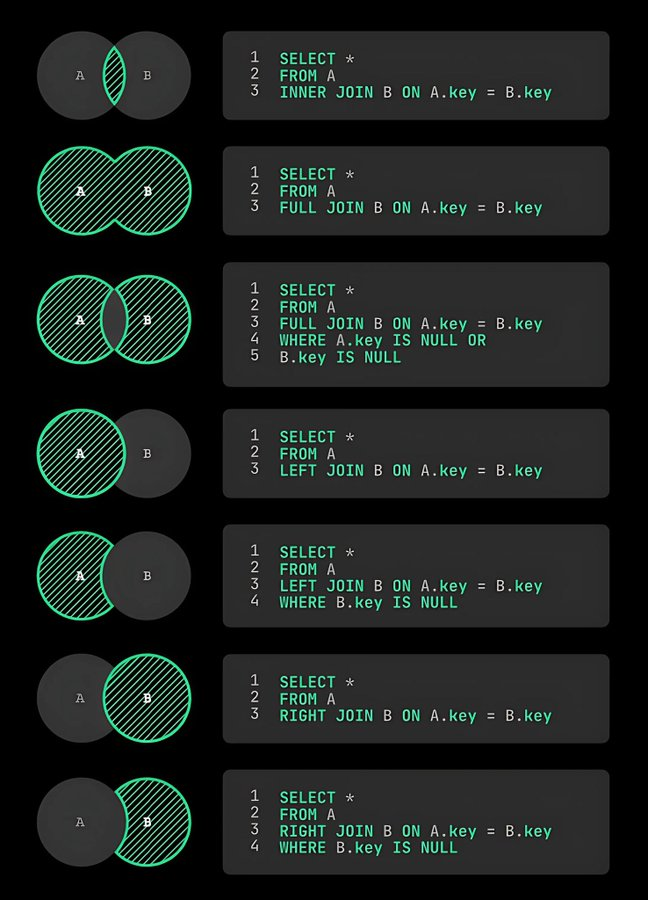
Finally
SQL joins are powerful tools that enable you to retrieve and combine data from multiple tables. Whether you're working with simple relationships or complex datasets, understanding how to use INNER JOIN, OUTER JOIN, and other types of joins will help you write more efficient and accurate SQL queries.
Remember, performance and the correct choice of join type are key to writing effective queries. By understanding the different types of joins and when to use them, you'll be able to unlock the full potential of relational databases and get the most out of your data.
Comments ()